- Web development
Simple cookie bar
EU cookie legislation requires website owners to inform visitors about the use of cookies. In this article, I will provide you with a simple solution of an informative cookie bar.
What it does
When a page gets loaded, a message shows up. If the visitor closes the message, a localStorage flag gets set, and the message won´t display any more. See the example.
The code
The solution consists of 2 parts:
- logic based on Javascript and
- styling.
Both parts need to be implemented in the website to make it work. For javascript, you can find jQuery and vanilla implementations below.
For styling, you can choose from CSS or LESS implementations.
Please, note that the javascript code relies on the existence of the .footer
element. You can, of course, change it according to your taste.
jQuery
$(function () {
if (localStorage) {
if (localStorage.getItem('CookieBarHide') !== 'true') {
var bar = '<div class="cookie-bar js-cookie-bar"><div class="cookie-bar__inner"><div class="cookie-bar__text">The website uses small cookies to improve your website experience. You may disable them from your browser settings at any time. <a href="#" target="_blank" rel="noopener noreferrer">Learn more</a>.</div><div class="cookie-bar__close js-cookie-bar__close">×</div></div></div>';
$('.footer').after(bar);
}
$('.js-cookie-bar__close').click(function () {
$(this).parents('.js-cookie-bar').remove();
localStorage.setItem('CookieBarHide', 'true');
});
}
});
Vanilla javascript
(function () {
if (localStorage) {
if (localStorage.getItem('CookieBarHide') !== 'true') {
var bar = '<div class="cookie-bar js-cookie-bar"><div class="cookie-bar__inner"><div class="cookie-bar__text">The website uses small cookies to improve your website experience. You may disable them from your browser settings at any time. <a href="#" target="_blank" rel="noopener noreferrer">Learn more</a>.</div><div class="cookie-bar__close js-cookie-bar__close">×</div></div></div>';
document.querySelector('.footer').insertAdjacentHTML('afterend', bar);
}
var closeEl = document.querySelector('.js-cookie-bar__close');
if (closeEl !== null) {
closeEl.addEventListener('click', function () {
var barEl = document.querySelector('.js-cookie-bar');
barEl.parentNode.removeChild(barEl);
localStorage.setItem('CookieBarHide', 'true');
});
}
}
})();
CSS
.cookie-bar {
position: fixed;
bottom: 0;
left: 0;
width: 100%;
background-color: #a3bf3b; /*Bar bg color*/
z-index: 999;
padding: 5px 0;
line-height: 1.4em;
}
.cookie-bar__inner {
color: #fff; /*Bar text color*/
text-align: left;
margin-right: auto;
margin-left: auto;
padding-left: 10px;
padding-right: 10px;
position: relative;
}
/*Bar wrapper width*/
@media (min-width: 1000px) {
.cookie-bar__inner {
width: 1000px;
}
}
.cookie-bar__inner a {
color: #fff; /*Bar link color*/
}
.cookie-bar__text {
padding-right: 8px;
display: inline-block;
width: 92%;
}
.cookie-bar__close {
color: #fff; /*Bar close icon color*/
font-size: 28px;
cursor: pointer;
position: absolute;
top: 0;
right: 10px;
}
LESS
.cookie-bar {
position: fixed;
bottom: 0;
left: 0;
width: 100%;
background-color: #a3bf3b; /*Bar bg color*/
z-index: 999;
padding: 5px 0;
line-height: 1.4em;
&__inner {
color: #fff; /*Bar text color*/
text-align: left;
margin-right: auto;
margin-left: auto;
padding-left: 10px;
padding-right: 10px;
position: relative;
/*Bar wrapper width*/
@media (min-width: 1000px) {
width: 1000px;
}
a {
color: #fff; /*Bar link color*/
}
}
&__text {
padding-right: 8px;
display: inline-block;
width: 92%;
}
&__close {
color: #fff; /*Bar close icon color*/
font-size: 28px;
cursor: pointer;
position: absolute;
top: 0;
right: 10px;
}
}
About the author
Milan Lund is a Freelance Web Developer with Kentico Expertise. He specializes in building and maintaining websites in Xperience by Kentico. Milan writes articles based on his project experiences to assist both his future self and other developers.
Find out more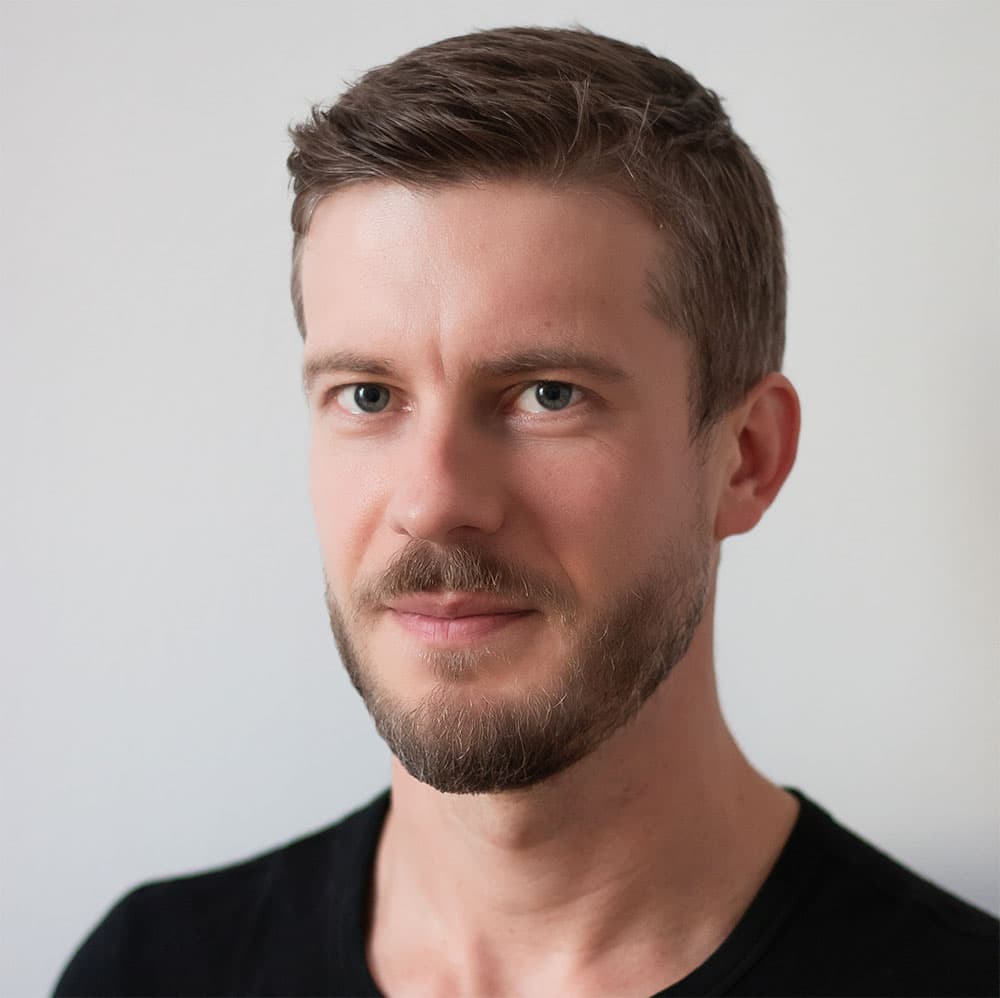