- Kentico CMS
Custom macro: Get the current user's country and state
In this post I will provide you with a code example of a custom macro that gives you country and state of the current user.
The problem
My client asked me to display country and state of authenticated users on his Kentico website. The information is stored in the UserCountry
column of the CMS_User
table. Regrettably, the names are stored in the codename format so it is not possible to show them directly with use of the {%CurrentUser.UserCountry%}
macro. You would end up with results like AntiguaAndBarbuda
or USA;Louisiana
.
Initially, I came up with a macro that returns display name of the current user's country: {% GlobalObjects.Countries.Where("CountryName = '" + CurrentUser.UserCountry + "'"+).FirstItem.CountryDisplayName %}
. Unfortunatelly, the macro ignores states. It displays country names as you would expect i.e. "Antigua and Barbuda" but for USA it returns nothing.
Custom macro is the solution
It would be painful to come up with a macro that takes states into account in Portal Engine. I decided to create a custom macro with use of the Custom macro fields. See the documentation for extending the Kentico macro engine by adding custom macro fields. Below you can see full code for the macro with comments. See the getUserCountryName
method if you are interested only in the logic of getting countries and states. With the code below you can use the {% UserCountryName %}
macro in the Portal Engine.
using CMS;
using CMS.DataEngine;
using CMS.MacroEngine;
using CMS.Membership;
using CMS.Globalization;
// Registers the custom module into the system
[assembly: RegisterModule(typeof(CustomMacroModule))]
public class CustomMacroModule: Module {
// Module class constructor, the system registers the module under the name "CustomMacros"
public CustomMacroModule(): base("CustomMacros") {}
// Contains initialization code that is executed when the application starts
protected override void OnInit() {
base.OnInit();
// Adds the 'UserCountryName' field to the global macro resolver
MacroContext.GlobalResolver.SetNamedSourceDataCallback("UserCountryName", getUserCountryName);
}
// Callback method that defines the result of the 'UserCountryName' macro field
private object getUserCountryName(EvaluationContext context) {
string countryName = "";
// Gets the current user
UserInfo user = UserInfoProvider.GetFullUserInfo(MembershipContext.AuthenticatedUser.UserID);
// Checks that the user exists
if (user != null) {
// Get user country
countryName = user.GetValue("UserCountry", "");
// Splits the country and gets country and state code names
string[] countryState = countryName.Split(';');
// Gets country
CountryInfo country = CountryInfoProvider.GetCountryInfo(countryState[0]);
if (country != null) {
// Gets country name
countryName = country.CountryDisplayName;
// Is there a state as well
if (countryState.Length > 1) {
// Get state
StateInfo state = StateInfoProvider.GetStateInfo(countryState[1]);
// Compose full coutry and state output
if (state != null) {
countryName += ", " + state.StateDisplayName;
}
}
}
}
return countryName;
}
}
About the author
Milan Lund is a Full-Stack Web Developer, Solution Architect, and Consultant for Xperience by Kentico projects, working as both a freelancer and a contractor. He specializes in building and maintaining websites using Xperience by Kentico. Milan writes articles based on his project experiences to assist both his future self and other developers.
Find out more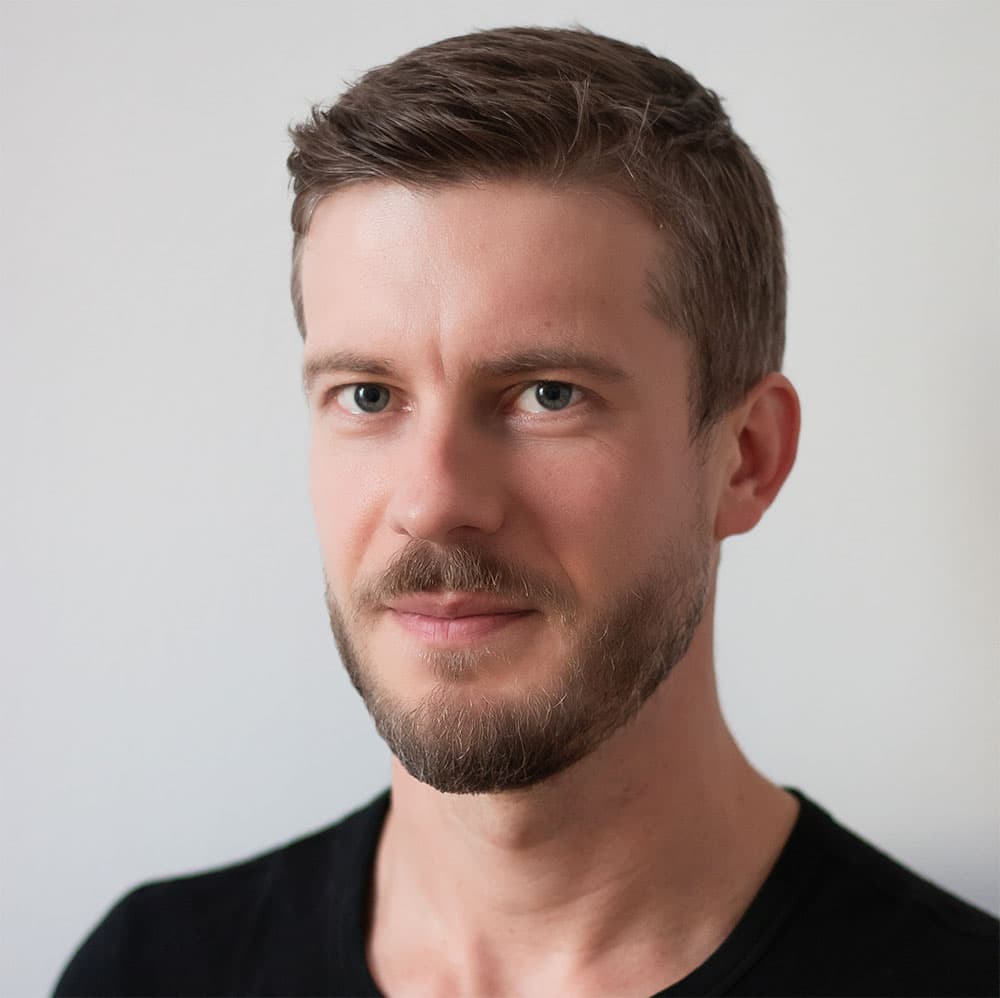